The UK will have to hold European elections,despite hopes from the government a Brexit deal would be done by then, says the PM’s de facto deputy. The vote is due on 23 May, but Theresa May said the UK would not have to take part if MPs agreed a Brexit plan first. The UK was due to leave the EU on 29 March, but as no deal was agreed by Parliament, the EU extended the deadline to 31 October. It can leave the bloc earlier, but if the UK has not left by 23 May, it is legally obliged to take part in the EU-wide poll and to send MEPs to Brussels.
UK will take part in European elections
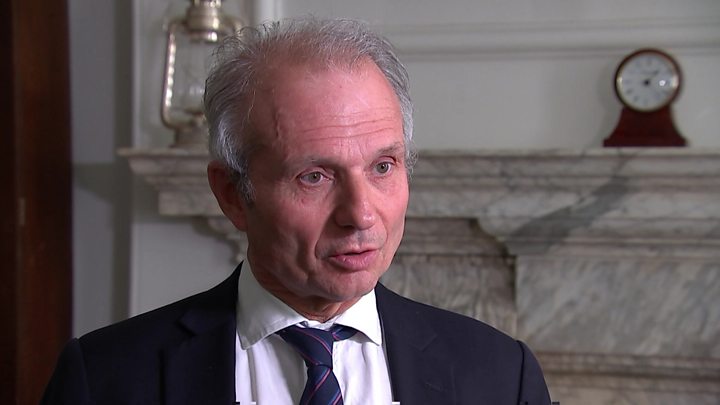